A Simple bash script to modify macOS DNS by detecting SSID
I believe most of us have different DNS settings for different networks. For example, in my case, I use Google's public DNS `8.8.8.8` and C
Read MoreMendix: How to debug javaaction with IntelliJ and VS Code
- 14 Jul, 2024
Sometimes, you may want to debug the Java actions in Mendix project. In the Mendix Documentation, it only shows how to debug Java actions w
Read MoreMendix: How to connect to built in hsql database from dbeaver
- 29 Aug, 2023
By default, Mendix project is using HSQL as the database.If you want to check the db tables and data, you can simply do this:- start th
Read MoreFixing the Close Action for a Modal popup in Mendix
- 01 Aug, 2023
Our tester engineer recently reported an issue where in a Mendix app, it takes more than 3 seconds to close a popup after clicking the clos
Read MoreMendix: How to call Microflow in Java Action and use its return value
- 28 Oct, 2022
After you dive into Mendix for a longer time, you would find yourself writing java code now and then. Don't worry, good news is that you do
Read More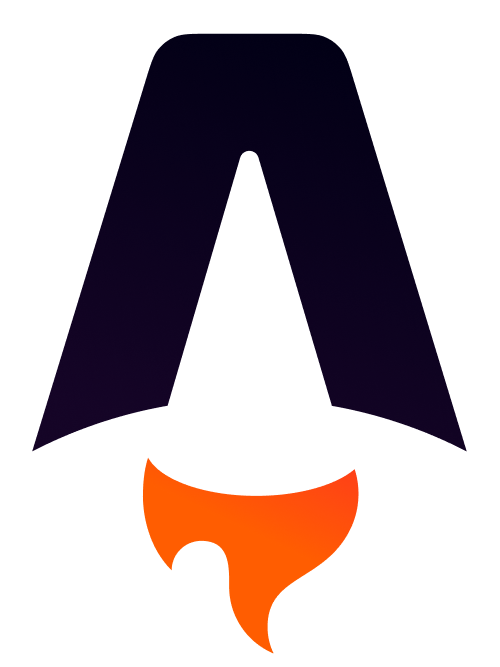
I am available for freelance work
I am available for freelance work. If you have a project that you want to get started, think you need my help with something or just fancy saying hey, then get in touch.
Send me a message